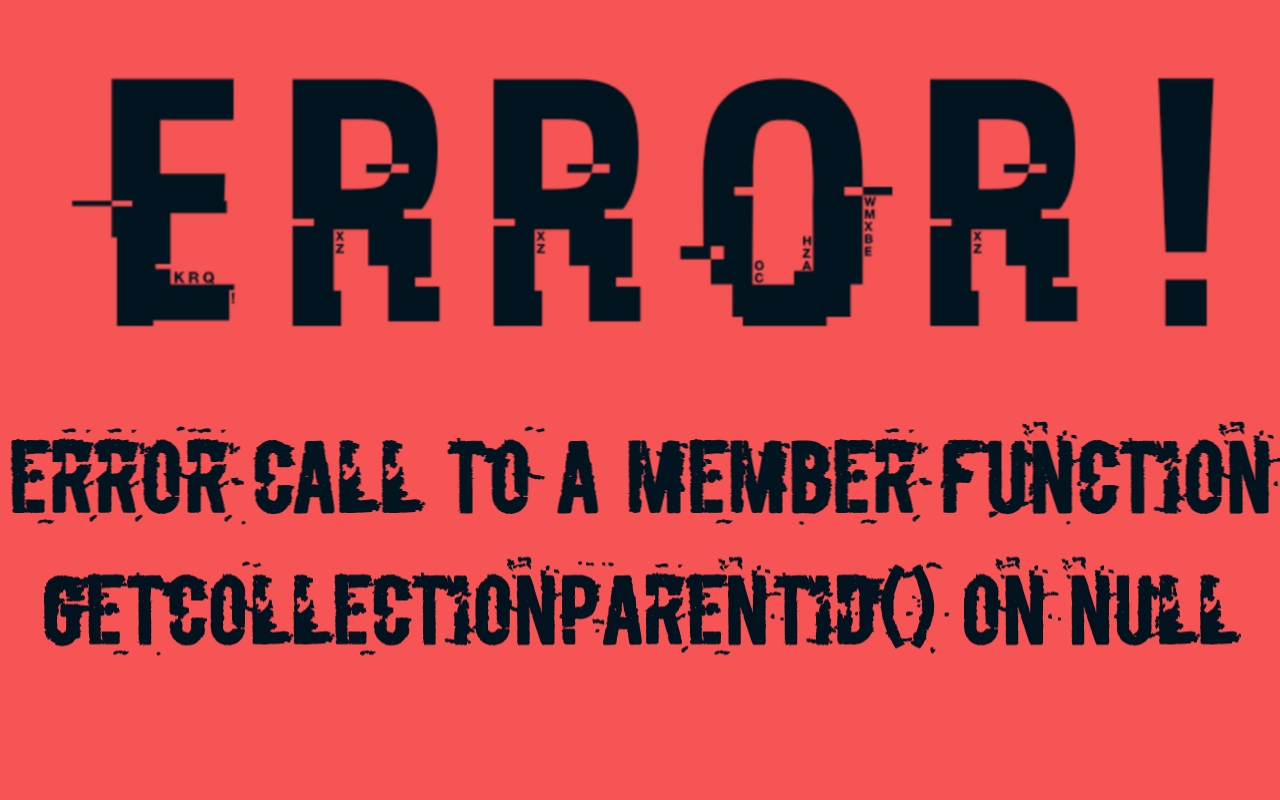
Error Call to a Member Function getcollectionparentid() on Null
Errors during software development are inevitable, especially when working with complex systems or object-oriented programming. One common runtime error faced by developers is “Call to a member function getcollectionparentid()
on null.” This error arises when the code tries to invoke a method (getcollectionparentid()
) on an object that is unexpectedly null
. It often stems from logical oversights, unhandled edge cases, or data retrieval failures.
In this comprehensive guide, we will discuss the possible causes of this error, real-world examples, methods for diagnosis, and robust solutions to prevent its recurrence. Whether you are a seasoned developer or a beginner, this guide will provide you with actionable insights to handle this issue efficiently.
Table of Contents
ToggleWhat Does the Error “Call to a Member Function getcollectionparentid()
on Null” Mean?
The error “Call to a member function getcollectionparentid()
on null“ essentially means that the code attempted to call the getcollectionparentid()
method on an object that was either:
- Not instantiated (i.e., it doesn’t exist).
- Explicitly set to
null
.
In object-oriented programming (OOP), methods are tied to objects. If the object is null, invoking its methods is impossible, leading to this error. For instance:
$collection = null; // Object is null
echo $collection->getcollectionparentid(); // Runtime error
Here, $collection
is null, so calling the method getcollectionparentid()
on it triggers the error.
Common Scenarios Where This Error Occurs
This error can appear in various contexts, especially in applications involving database interactions, API calls, or hierarchical data structures. Let’s explore some common situations:
1. Uninitialized Objects
When an object is declared but not assigned a value, it defaults to null
. Attempting to call a method on such an uninitialized object will trigger this error.
Example:
$collection;
echo $collection->getcollectionparentid(); // Error
2. Failed Database Retrieval
In many applications, objects are loaded from databases. If the database query fails (e.g., due to a missing record), the object remains null.
Example:
$collection = $db->findCollectionById($id); // Returns null if not found
echo $collection->getcollectionparentid(); // Error
3. API Response Issues
When fetching data from an external API, missing or incomplete responses might leave an object uninitialized.
Example:
$response = $api->fetchCollectionData($id);
$collection = $response->collection ?? null;
echo $collection->getcollectionparentid(); // Error4. Invalid Parent-Child Relationships
In hierarchical data models (e.g., categories, directories), a missing or invalid parent can cause null objects, leading to this error.
Example:
$parent = $child->getParentCollection();
echo $parent->getcollectionparentid(); // Error if $parent is null
Root Causes of the Error
To resolve this error effectively, it’s essential to understand its underlying causes. Below are some common reasons why this error occurs:
1. Logic Errors in Code
Sometimes, the code assumes that an object will always exist under certain conditions. When those conditions are not met, the object remains null, causing the error.
2. Data Retrieval Failures
Objects retrieved from databases, APIs, or other sources may fail due to incorrect queries, missing records, or connectivity issues.
3. Unverified Return Values
Functions or methods that are supposed to return objects may return null in specific cases, such as when no matching data is found.
4. Improper Dependency Management
Dependencies such as database connections, APIs, or configuration settings might not be initialized properly, leading to null objects.
5. Unmanaged Edge Cases
Developers might overlook scenarios where data is missing or invalid, leaving objects uninitialized.
Diagnosing the Error
When this error appears, systematic debugging is the key to identifying its root cause. Here are some effective ways to diagnose the issue:
1. Check Object Initialization
Trace the point where the object is created or assigned. Verify whether it was initialized properly before the method call.
Example Debugging Code:
if ($collection === null) {
error_log("Collection is null at this point.");
}
2. Log Database Queries
If the object is retrieved from a database, log the query and its result to ensure it is functioning as expected.
Example:
$query = "SELECT * FROM collections WHERE id = $id";
$result = $db->executeQuery($query);
if (empty($result)) {error_log(“No data found for ID: “ . $id);
}
3. Use Debugging Tools
Use debugging tools to inspect the object’s state at runtime. In PHP, tools like Xdebug can help visualize the flow of data and object initialization.
4. Validate Input Data
Ensure that input data used to retrieve or create the object is valid and complete.
Solutions to Fix the Error
1. Add Null Checks
Before calling the method, verify if the object is null. This is a simple yet effective way to prevent the error.
Example:
if ($collection !== null) {
echo $collection->getcollectionparentid();
} else {
echo "The collection is null.";
}
2. Set Default Values
In scenarios where null objects are possible, provide a default value or object to avoid runtime errors.
Example:
$collection = $db->findCollectionById($id) ?? new DefaultCollection();
echo $collection->getcollectionparentid();
3. Error Handling with Try-Catch
Wrap the code in a try-catch block to handle errors gracefully.
Example:
try {
echo $collection->getcollectionparentid();
} catch (Exception $e) {
error_log($e->getMessage());
echo "An error occurred.";
}
4. Ensure Object Initialization
Ensure that objects are properly instantiated and initialized before they are used.
Example:
$collection = new Collection($data);
echo $collection->getcollectionparentid();
5. Refactor Code Logic
Revisit the code logic to address scenarios where objects might remain null. This may involve restructuring database queries, API calls, or data validation.
Best Practices to Prevent the Error
Adopting best practices can significantly reduce the chances of encountering this error:
1. Defensive Programming
Always anticipate potential issues, such as null objects, and write code to handle them proactively.
2. Type Declarations
Use type declarations in your code to enforce object types and avoid null values where possible.
Example:
function getCollection(Collection $collection): string {
return $collection->getcollectionparentid();
}
3. Comprehensive Testing
Test your code thoroughly, especially for edge cases where objects might be null.
4. Clear Documentation
Document methods and their return values, specifying scenarios where null might be returned.
Real-World Examples
Example 1: Hierarchical Data in Content Management Systems
In CMS platforms, content is often organized hierarchically. Missing parent content can lead to null objects.
Example Fix:
$parent = $collection->getParent();
if ($parent !== null) {
echo $parent->getcollectionparentid();
} else {
echo "Parent collection is missing.";
}
Example 2: Product Categories in E-commerce Applications
In e-commerce, product categories may reference parent categories. If a parent category is deleted, it could result in null objects.
Example Fix:
$parentCategory = $category->getParentCategory();
if ($parentCategory) {
echo $parentCategory->getcollectionparentid();
} else {
echo "No parent category available.";
}
Conclusion
The “Call to a member function getcollectionparentid()
on null” error highlights a common challenge in programming: ensuring that objects are properly initialized and validated before use. By understanding its causes, employing robust debugging methods, and following best practices, developers can effectively prevent and resolve this issue.
Whether working with databases, APIs, or hierarchical data, handling null objects gracefully is a critical skill that enhances code reliability and reduces runtime errors.